In this article I explain how to connect Enigma Protector with payment processing system Verifone to automatically generate license keys for protected files if customer orders product online using Verifone.
Shortly, if you are new in all of this, what is Verifone? Verifone is a service that allows software developers to sell own products for end customers, without any knowledge of payment processing systems, how money can be transferred from end customer to developer, no dealing with VAT, invoices etc, this everything is being handled by Verifone.
Here we will assume that developer sells products on Verifone, products are protected with Enigma Protector, and after successful order the end customer has to receive license key in order to use protected software. In additional, we recommend developers to divide public and private versions of software they sell, public version could be available at website as a DEMO, for anyone for testing (it could be even not protected by Enigma Protector, but has some limited functionality to force customers to order commercial version), private version is protected with Enigma Protector and is being delivered to customer after an order.
We provide a separate product Online Activation Panel that allows integration with payment processing systems, including Verifone, that is combined with the Enigma Protector and has flexible interface for managing of incoming orders, customers, activations etc.
Online Activation Panel for sales automation
Quick guide how to protect private version (the one that end customer receives after the order is made) in Enigma Protector, just few steps for basic protection:
- Generate new project in Enigma Protector using main menu – Files – New Project. This is mandatory!
- On the Input panel select the file you wish to protect, set unique product name and version
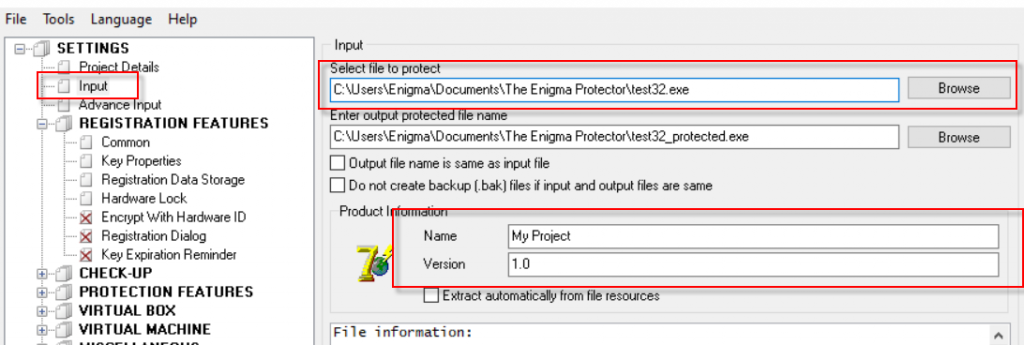
- Enable the option Registration Features – Common – Allow execution only if registered, so it will be impossible to run protected file without license key
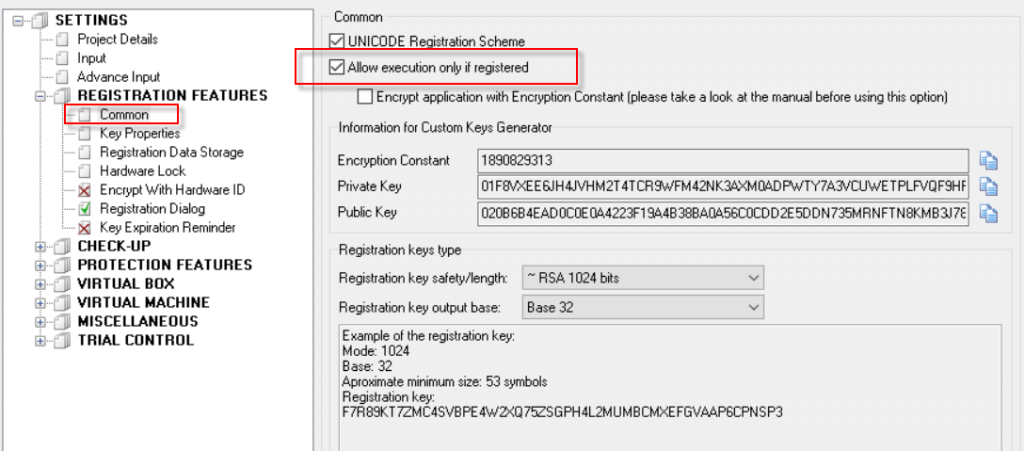
- Enable Registration Features – Registration Dialog option in order to show customers a form to enter license key
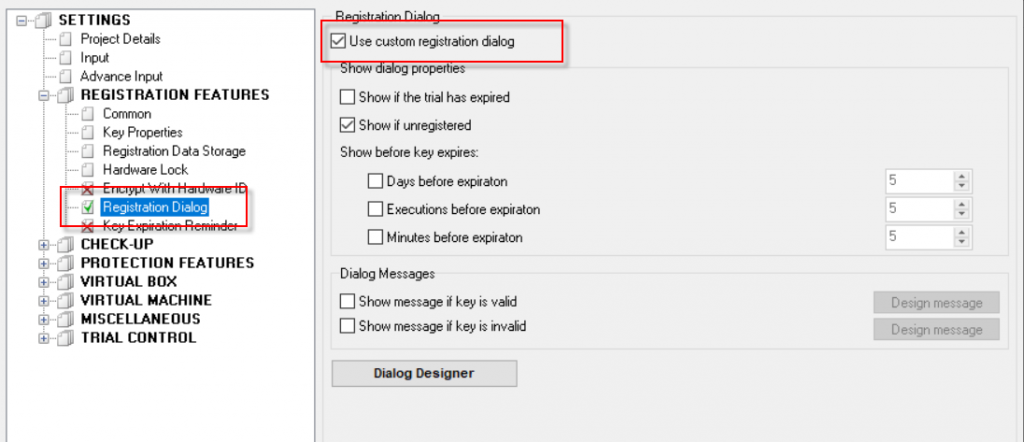
- I also suggest, in this particular case, make a small change to the registration dialog. Click the Dialog Designer button, select the label with text “Name” and change it’s caption property to “Email”. What here I mean is the following. Licensing, in Enigma Protector performs using a pair of registration name plus registration key. Due to specific of Verifone platform we are unable to setup custom registration name, so let’s use end customer’s email address as a registration name. After customer places an order and receive license key, he/she will need to enter email address that was used to create an order and the license key received by Verifone in the Enigma Protector registration dialog. It is also reasonable to remove Try button, since in the private version we do not provide an ability to try protected application
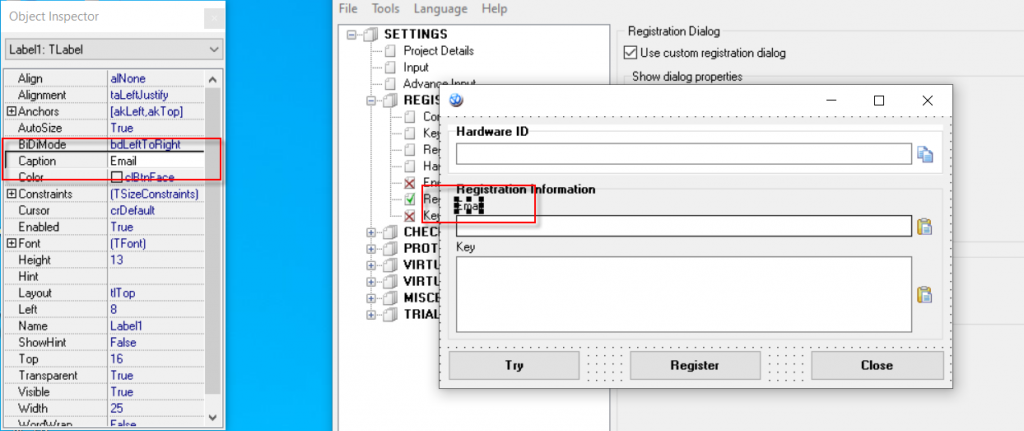
- Next you can protect the file, run it and see the following dialog, asking to enter license information, that customer will receive after an order
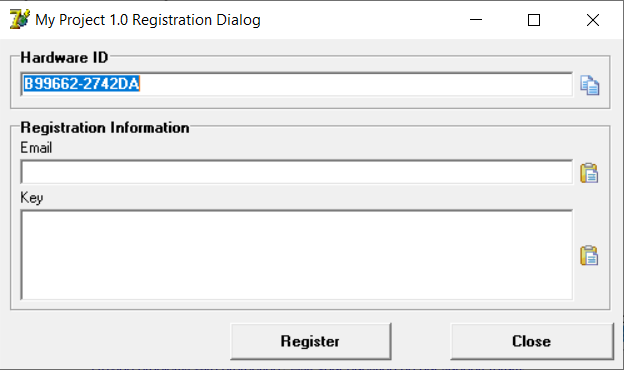
OK, at this step we are done with protector.
For the next step we need to develop a PHP script, that will be integrated to Verifone, that will generate license key for Enigma Protector. As a code template we will use the PHP code sample provided by Verifone at https://verifone.cloud/docs/2checkout/API-Integration/Webhooks/API-Message-Services/Key-generators
We will use, so called, IPN (Instead Payment Notification) system to generate license key. The purpose of IPN is to notify custom script that new order comes and pass order parameters, i.e. Verifone will call our PHP script when new order comes, this moment we will generate license key for protected file, then Verifone delivers registration key to end customer, so he/she can enter it to the program to register.
There is a script, that I recommend to use for generating of license key:
<?php
// We assume that the url of this script is private and known by software developer only.
// Otherwise, anyone will be able to use it to generate license keys for protected software
// As a basic template for this keys generator we are using PHP code provided by Verifone
// https://verifone.cloud/docs/2checkout/API-Integration/Webhooks/API-Message-Services/Key-generators
function stripslashes_recursive(&$value, $key){
$value = stripslashes($value);
}
function strip_magic_quotes($data){
if (ini_get('magic_quotes_gpc') ){
if(is_array($data)){
array_walk_recursive($data, 'stripslashes_recursive');
reset($data);
}
else{
$data = stripslashes($data);
}
}
return $data;
}
function generate_key_post($url, $data) {
$ch = curl_init();
// set URL and other appropriate options
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// grab URL and pass it to the browser
$response = curl_exec($ch);
if (curl_errno($ch)) {
return '';
}
// close cURL resource, and free up system resources
curl_close($ch);
return $response;
}
//some servers still have magic_quotes_gpc on
//to validate the hash we need the information 2Checkout sent, unaltered by client server
$cleaned_post = strip_magic_quotes($_POST);
//rudimentary example of business logic
//this example uses only 2Checkout ProductId to decide the output
switch($cleaned_post['PID'])
{
case '123456789': //for this product your key is a string
// Generate license key for Enigma Protector
// Use email address as a registration name for the key
$data['Action'] = 'GenerateKeyW';
$data['KeyMode'] = 1024;
$data['KeyBase'] = 32;
$data['Hyphens'] = 1;
$data['EncryptedConstant'] = 1890829313;
$data['PrivateKey'] = '01F8VXEE6JH4JVHM2T4TCR9WFM42NK3AXM0ADPWTY7A3VCUWETPLFVQF9HF6PCUFUSFGMXWXU38SAY8SJDEMEZAY3TJSEE898GGV32475PBCGZJKKNXRCZZ4DHVSL3XMX2ZJRLK8KUWZLNSPLZ9K8AAFMZ4QY7C9RJH64XL9TFHZJT342TXQTV8DHTHB2PHBQVAVGSHKU5ANCYTCRE020497F7ZRC7ZMMTRREMECBWB7EKQ7Z9B4X020497F7ZRC7ZMMTRREMECBWB7EKQ7Z9B4X';
$data['PublicKey'] = '020B6B4EAD0C0E0A4223F19A4B38BA0A56C0CDD2E5DDN735MRNFTN8KMB3J78P26A5R6RUQ6TTV3TMQ5U2EQMKUKUWB9VGFVBX22KYS822LXFMXGY65ZBA2ZDDAW6FH3T4AC8CLB59KFKTZYHZPCVNZGKFCZ77XRVDBBJ82EH4VKX2VE5FC7WPVJAVGWW7QP8UYN657PZUMPM3VX59FQRH9LXSR2N2YFS8VTVGCZULWUYUWLSP0CDK4TACJLUBWFWQZKVTXH7C7EGLWP77P8FD6DP7LLYUEW7Z8A3G5CMH9YQDVCTR3ZVKHJXUMTHGQZ3F3G2677FUUSMB6ZHSUMR39FH2XAE39TKCSRLJY9N8RZHYE7K8A9W58GJ9JPKV6QNP46Z7HS7HQ7UTS99NHG7H8H3YFDB9G83TXKNF2WNUQVKTTGXFFTQCTWGN3A37BSYL0CDMSKZ7Y7Y778G5GK4VC6N568F9MQJT3URNLVSVP78KXLDGNRDN5NH4CESQSJEJVCQ4NWGZQM6ULJYA7A4WULQGC9FZMU4LXZMK9Y32PP4F4A872GUGX83CUEN5GWD77CDPBAKUUUF69BKV6VKBKMX6ML2JP3GRKVPFED2VGMJWZ7LRDFC5RRAVGX3QMYUG4HD6P2CTHVSJUBFX';
$data['RegName'] = $cleaned_post['EMAIL'];
$regkey = generate_key_post('https://enigmaprotector.com/cgi-bin/keygen', $data);
if ($regkey) {
//build a basic xml with the following structure:
$basic_xml = '<?xml version="1.0"?>
<data>
<code>'.$regkey.'</code> <!-- your string key here -->
</data>';
header('HTTP/1.0 200 OK',false, 200); //inform 2Checkout you received a valid post, you shouldn't have to set this header explicitly
header('Content-Type: text/xml');
echo $basic_xml; //make sure the script doesn't output anything after this point and/or stop script execution
}
exit;
break;
}
Some comments to the code above:
- Script prepares array of data to send to CGI keys generator. The array contains values from Enigma Protector project’s file, basically information from Registration Features – Common panel. These values are: PrivateKey, PublicKey, EncryptionConstant, KeyMode and KeyBase. The Action value depends on a Unicode checkbox of registration settings, if it is checked, then Action should be GenerateKeyW, if not checked then GenerateKey
- CGI keys generator for Windows and Linux servers can be found at Enigma Protector’s installation folder, Examples\Keygen\CGI subfolder. We recommend to setup CGI keygen on own server (use recommendations from manual https://enigmaprotector.com/en/help/manual/81ffd9d0173d967561bcf5f6ec7d697d) or use preinstalled on our server https://enigmaprotector.com/cgi-bin/keygen
- PID – product identifier can be found at Verifone’s cPanel, under Setup – Products page
Put above PHP script on own server, at some hidden path, so that nobody else, except Verifone, can use it to generate keys.
We are done with script that generates license keys, let’s configure Verifone, also from beginning with the basic scheme.
- Open Verifone’s cPanel, setup a new product under Setup – Products menu
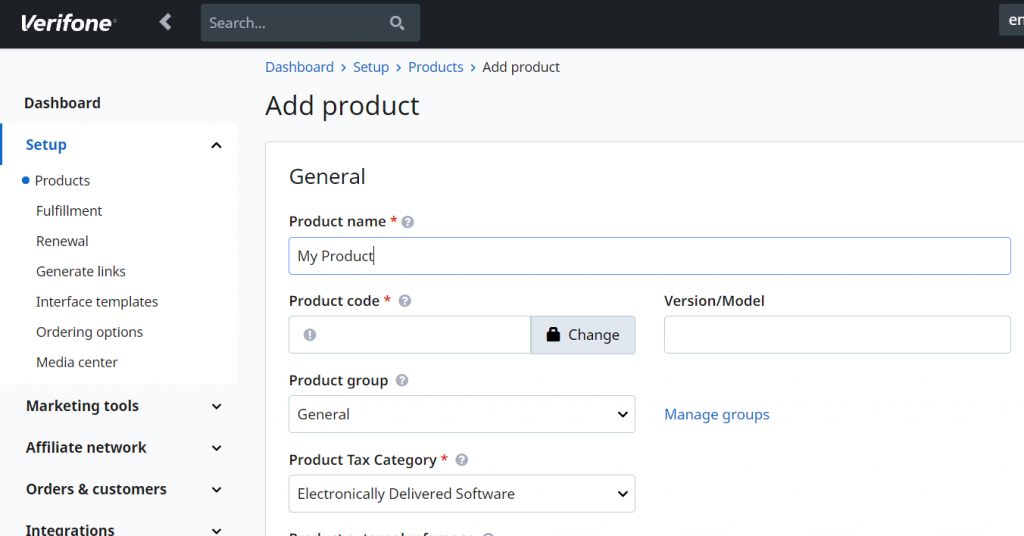
- Under Setup – Fulfillment we need to create a list of codes for created product. Enter list name and select the type to Dynamic
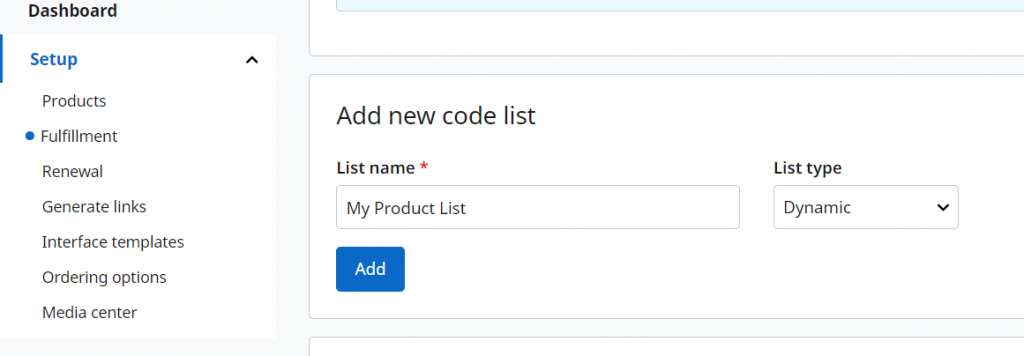
- Next step – enter url of the PHP script we just created. So Verifone will call this PHP script when new order comes. Click Set URL button
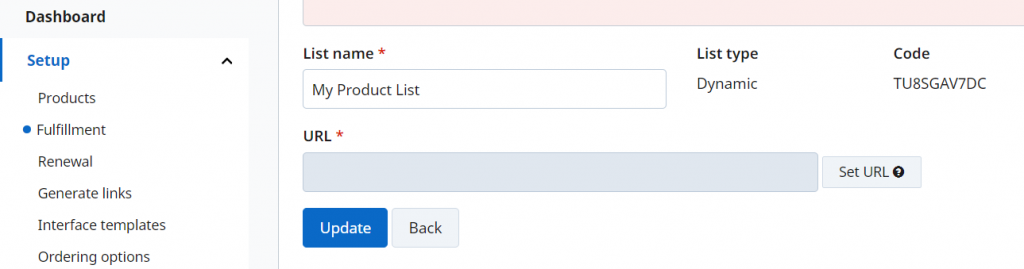
- Next we have to enter script url, test order parameters and test if it works
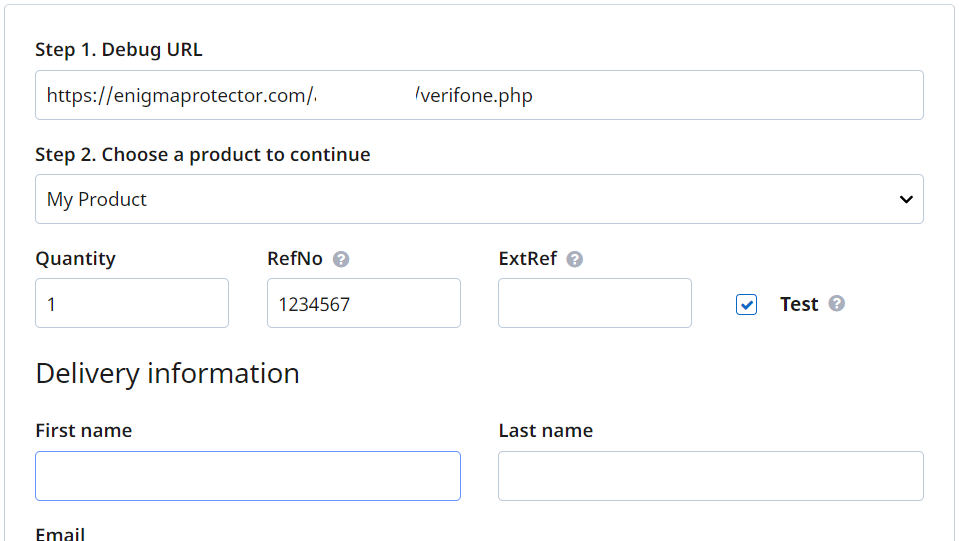
- If everything went well, then we have to see the successful message from Verifone, like this one
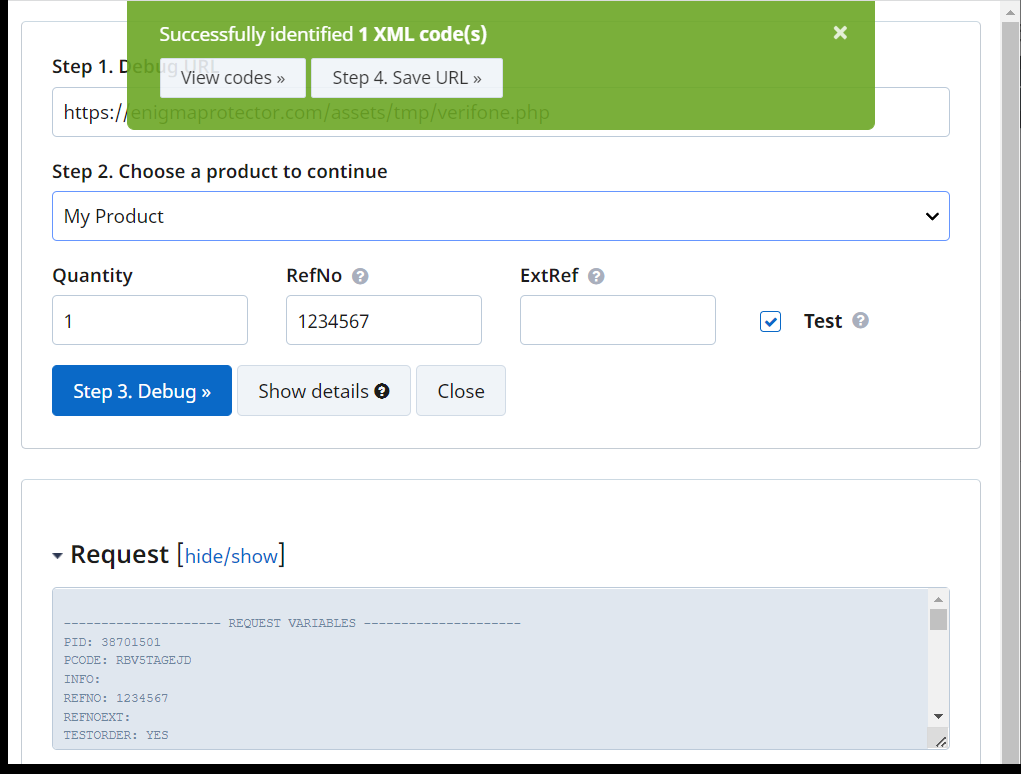
After all is done, we have fully working and automated scheme of software purchasing.
However, there are still some questions, not described here, but useful for developers. If you need anything of this described more detailed, please write us back at support@enigmaprotector.com and we append this tutorial.
- When create a product in Verifone, set the download url to the protected software, so that Verifone sends this link to end customer after purchase
- License keys, generated using this scheme, are not locked to particular computer. In order to lock the key to specific computer, the Hardware ID should be used. It can be specified by end customer in custom order field of Verifone order form and then be added to CGI keys generator through Hardware parameter. Btw, using our product Online Activation Panel this case can be handled automatically.
As noted above, we provide a separate product called Online Activation Panel it provides functions for integration with Verifone “from the box”, without any complex configurations or scripts. Online Activation Panel also allows to perform online activation with license keys that are tied to particular computer. If some solid solution for sales automation is required, then we recommend to take a look at Online Activation Panel.